|
|
|

|

|
Dicas
|

|
Visual Basic (Operações Matemáticas)
|
|
 |
Título da Dica: TRIGONOMETRIA
|
 |
|
|
Postada em 9/9/2003 por Ð@®l@n
1- ARCO COSENO
Function ArcCos(x As Double) As Double ' Inverse Cosine If x = 1 Then ArcCos = 0 ElseIf x = -1 Then ArcCos = -PI() Else ArcCos = Atn(x / Sqr(-x * x + 1)) + PI() / 2 End If End Function
2- ARCO SENO
Function Arccosec(x As Double) As Double ' Inverse Cosecant Arccosec = Atn(x / Sqr(x * x - 1)) + (Sgn(x) - 1) * PI() / 2 End Function
3- ARCO TANGENTE
Function Arccotan(x As Double) As Double ' Inverse Cotangent Arccotan = Atn(x) + PI() / 2 End Function
4- ARCO SECANTE
Function ArcSec(x As Double) As Double ' Inverse Secant ArcSec = Atn(x / Sqr(x * x - 1)) + Sgn(Sgn(x) - 1) * PI() / 2 End Function
5- ARCO SENO
Function ArcSin(x As Double) As Double ' Inverse Sine If x = 1 Then ArcSin = PI() / 2 ElseIf x = -1 Then ArcSin = -PI() / 2 Else ArcSin = Atn(x / Sqr(-x * x + 1)) End If End Function
6- ARCO TANGENTE (ALTERNATIVA)
Function ATan2(x As Double, y As Double) As Double ' ' Returns the ArcTangent based on X and Y coordinates ' If both X and Y are zero an error will occur. ' ' The positive X axis is assumed to be 0, going poistive in the ' counterclockwise direction, and negative in the clockwise direction. If x = 0 Then If y = 0 Then ATan2 = 1 / 0 ElseIf y > 0 Then ATan2 = PI() / 2 Else ATan2 = -PI() / 2 End If ElseIf x > 0 Then If y = 0 Then ATan2 = 0 Else ATan2 = Atn(y / x) End If Else If y = 0 Then ATan2 = PI() Else ATan2 = (PI() - Atn(Abs(y) / Abs(x))) * Sgn(y) End If End If End Function Function Cosec(x As Double) As Double ' Cosecant Cosec = 1 / Sin(x) End Function
Function Cotan(x As Double) As Double ' Cotangent Cotan = 1 / Tan(x) End Function
Function Graus2Radiano(x As Double) As Double ' Graus to radians Graus2Radiano = x / 180 * PI() End Function
Function HArccos(x As Double) As Double ' Inverse Hyperbolic Cosine HArccos = Log(x + Sqr(x * x - 1)) End Function
Function HArccosec(x As Double) As Double ' Inverse Hyperbolic Cosecant HArccosec = Log((Sgn(x) * Sqr(x * x + 1) + 1) / x) End Function
Function HArccotan(x As Double) As Double ' Inverse Hyperbolic Tangent HArccotan = Log((x + 1) / (x - 1)) / 2 End Function
Function HArcsec(x As Double) As Double ' Inverse Hyperbolic Secant HArcsec = Log((Sqr(-x * x + 1) + 1) / x) End Function
Function HArcsin(x As Double) As Double ' Inverse Hyperbolic Sine HArcsin = Log(x + Sqr(x * x + 1)) End Function
Function HArctan(x As Double) As Double ' Inverse Hyperbolic Tangent HArctan = Log((1 + x) / (1 - x)) / 2 End Function
Function HCos(x As Double) As Double ' Hyperbolic Cosine HCos = (Exp(x) + Exp(-x)) / 2 End Function
Function HCosec(x As Double) As Double ' Hyperbolic Cosecant = 1/HSin(X) HCosec = 2 / (Exp(x) - Exp(-x)) End Function
Function HCotan(x As Double) As Double ' Hyperbolic Cotangent = 1/HTan(X) HCotan = (Exp(x) + Exp(-x)) / (Exp(x) - Exp(-x)) End Function
Function HSec(x As Double) As Double ' Hyperbolic Secant = 1/HCos(X) HSec = 2 / (Exp(x) + Exp(-x)) End Function
Function HSin(x As Double) As Double ' Hyperbolic Sine HSin = (Exp(x) - Exp(-x)) / 2 End Function
Function HTan(x As Double) As Double ' Hyperbolic Tangent = HSin(X)/HCos(X) HTan = (Exp(x) - Exp(-x)) / (Exp(x) + Exp(-x)) End Function
Function PI() As Double PI = Atn(1) * 4 End Function
Function Radiano2Grau(x As Double) As Double ' Radians to Degrees Radiano2Grau = x / PI() * 180 End Function
Function Sec(x As Double) As Double ' Secant ' This will choke at PI/2 and 3PI/2 radians (90 & 270 degrees) ' Sec = 1# / Cos(x) End Function
|
|
|
|
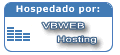
|